Skipping Tests
During the development process, there may be times when you need to temporarily disable a test. Rather than commenting out the code, we recommended using the skip()
method.
1it('has home', function () {2 //3})->skip();
When running your tests, Pest will inform you about any tests that were skipped.

You may also provide the reason for skipping the test, which Pest will display when running your tests.
1it('has home', function () {2 //3})->skip('temporarily unavailable');
In addition, there may be times when you want to skip a test based on a given condition. In these cases, you may provide a boolean value as the first argument to the skip()
method. This test will only be skipped if the boolean value evaluates to true
.
1it('has home', function () {2 //3})->skip($condition == true, 'temporarily unavailable');
You may pass a closure as the first argument to the skip()
method to defer the evaluation of the condition until the beforeEach()
hook of your test case has been executed.
1it('has home', function () {2 //3})->skip(fn () => DB::getDriverName() !== 'mysql', 'db driver not supported');
To skip a test on a particular operating system, you can make use of the skipOnWindows()
, skipOnMac()
, or skipOnLinux()
.
1it('has home', function () {2 //3})->skipOnWindows(); // or skipOnMac() or skipOnLinux() ...
Alternatively, you can skip a test on all operating systems except one by using onlyOnWindows()
, onlyOnMac()
, or onlyOnLinux()
.
1it('has home', function() {2 //3})->onlyOnWindows(); // or onlyOnMac() or onlyOnLinux() ...
Sometimes, you may want to skip a test on a specific PHP version. In these cases, you may use the skipOnPhp()
method.
1it('has home', function () {2 //3})->skipOnPhp('>=8.0.0');
The valid operators for the skipOnPhp()
method are >
, >=
, <
, and <=
.
Finally, you may even invoke the skip()
method within your beforeEach()
hook to conveniently skip an entire test file.
1beforeEach(function () {2 //3})->skip();
Creating Todos
While skipping tests can be a helpful way to exclude specific tests temporarily from your test suite, it can also lead to situations where skipped tests are forgotten or overlooked. To prevent this, Pest provide a way to create "todos", which are essentially placeholders for tests that need attention.
To begin working with todos, simply invoke the todo()
method.
1it('has home')->todo();
If you invoke the todo()
method on a test, Pest's output will inform you that the test is a todo so you don't forget about it.
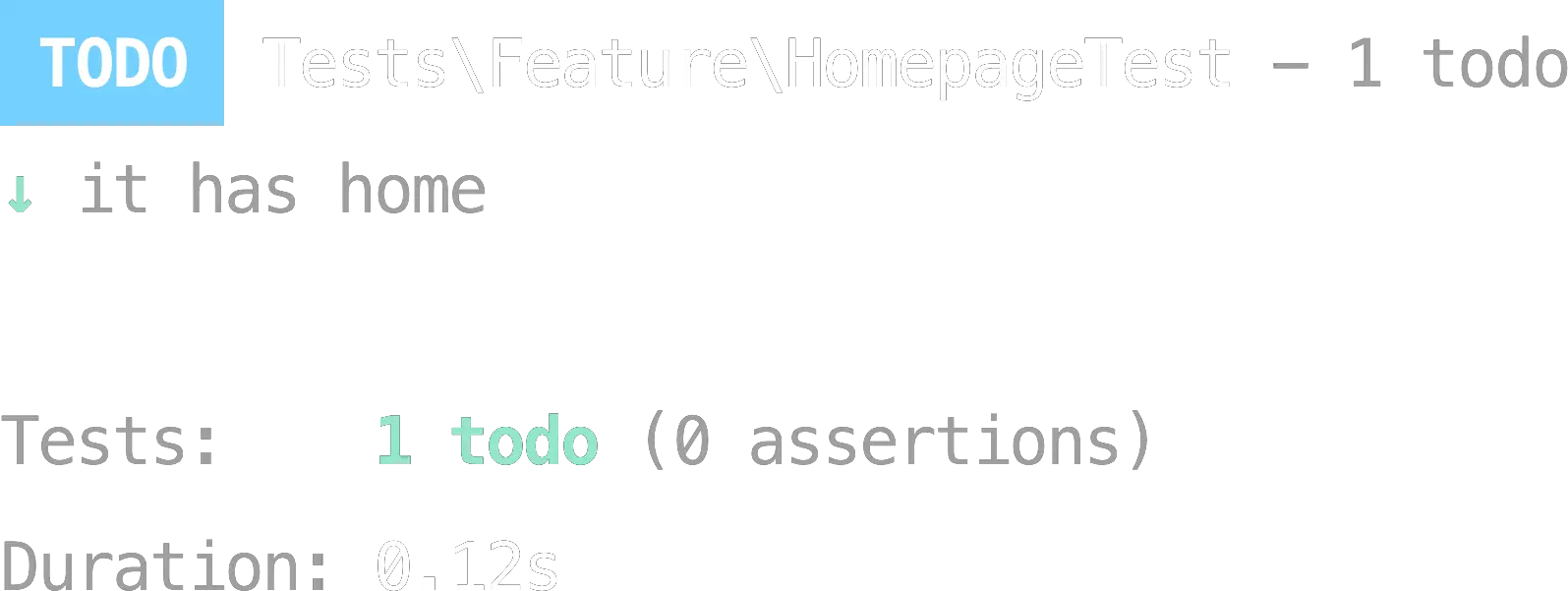
You can easily view a list of pending todos contained in your test suite by including the --todos
option when running Pest.
1./vendor/bin/pest --todos
As your codebase expands, it's advisable to consider enhancing the speed of your test suite. To assist you with that, we offer comprehensive documentation on optimizing your test suite: Optimizing Tests